Syntax Highlighting
Hugo support syntax highlighting natively1 using Chroma2 as its code highlighter.
Implementation
Implementation by Chroma
Hugo implements struct Highlighter
by importing chroma library.
The Highlight
methods is actually implemented in highlight
. We can summarize it to the following code:
|
|
Configuration in Hugo
Highlighting is carried out via the built-in highlight shortcode. It takes exactly one required parameter for the programming language to be highlighted and requires a closing shortcode. Note that highlight is not used for client-side JavaScript highlighting.
Options:
linenos
: configure line numbers. Valid values are true, false, table, or inline. false will turn off line numbers if it’s configured to be on in site config. New in v0.60.0 table will give copy-and-paste friendly code blocks.hl_lines
: lists a set of line numbers or line number ranges to be highlighted.linenostart=199
: starts the line number count from 199.anchorlinenos
: Configure anchors on line numbers. Valid values are true or false;lineanchors
: Configure a prefix for the anchors on line numbers. Will be suffixed with -, so linking to the line number 1 with the option lineanchors=prefix adds the anchor prefix-1 to the page.hl_inline
Highlight inside a(inline HTML element) tag. Valid values are true or false. The code tag will get a class with name code-inline. New in v0.101.0
Supported languages
Go
|
|
Java
|
|
Python
|
|
Shell
|
|
C
|
|
Cpp
|
|
Scala
|
|
Diff
|
|
JS
|
|
Kotlin
|
|
C-Sharp
|
|
Html
|
|
PHP
|
|
no named code block
## edit the file
$vi foo.md
+++
date = "2014-09-28"
title = "creating a new theme"
+++
bah and humbug
:wq
highlight shortcode
example:
{{< highlight go "linenos=table,hl_lines=8 15-17,linenostart=199" >}}
// ... code
{{< /highlight >}}
result:
|
|
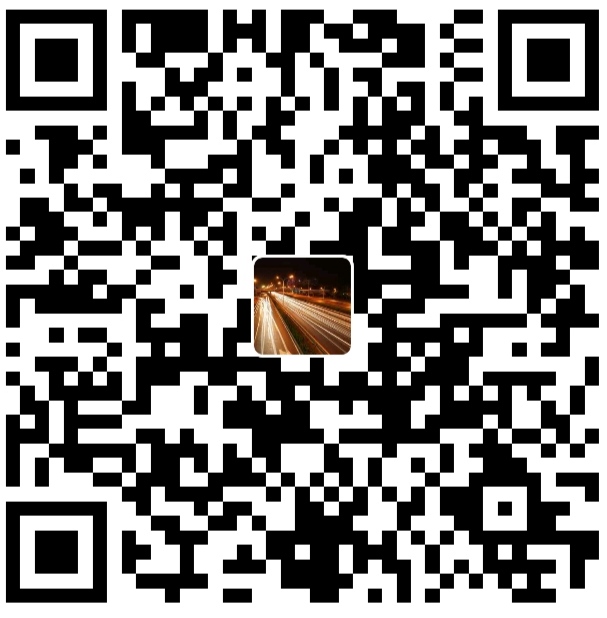
alipay
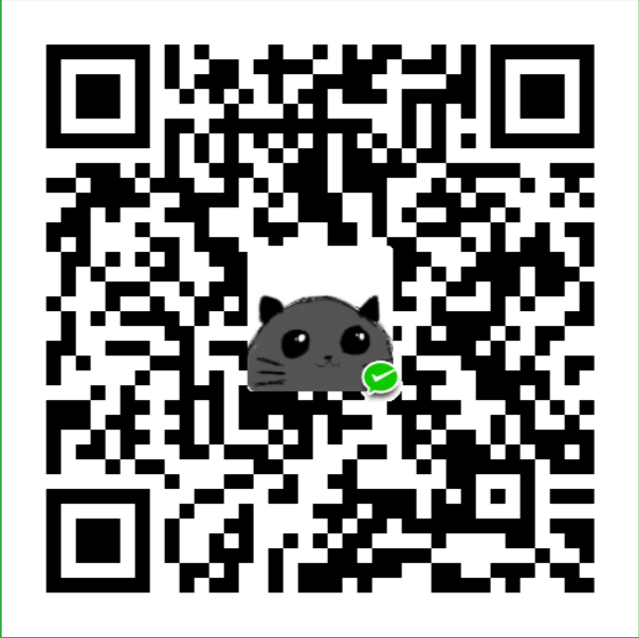
Author Houmin Wei
Publish August 30, 2022
LastMod July 11, 2023 (8d68658)
License 本作品采用 CC BY-NC-ND 4.0 许可协议进行许可,转载时请注明原文链接
如果你在浏览博客的过程中发现了任何问题,欢迎在对应文章下评论。如果你有其他事情想要咨询,可以通过邮件联系我。
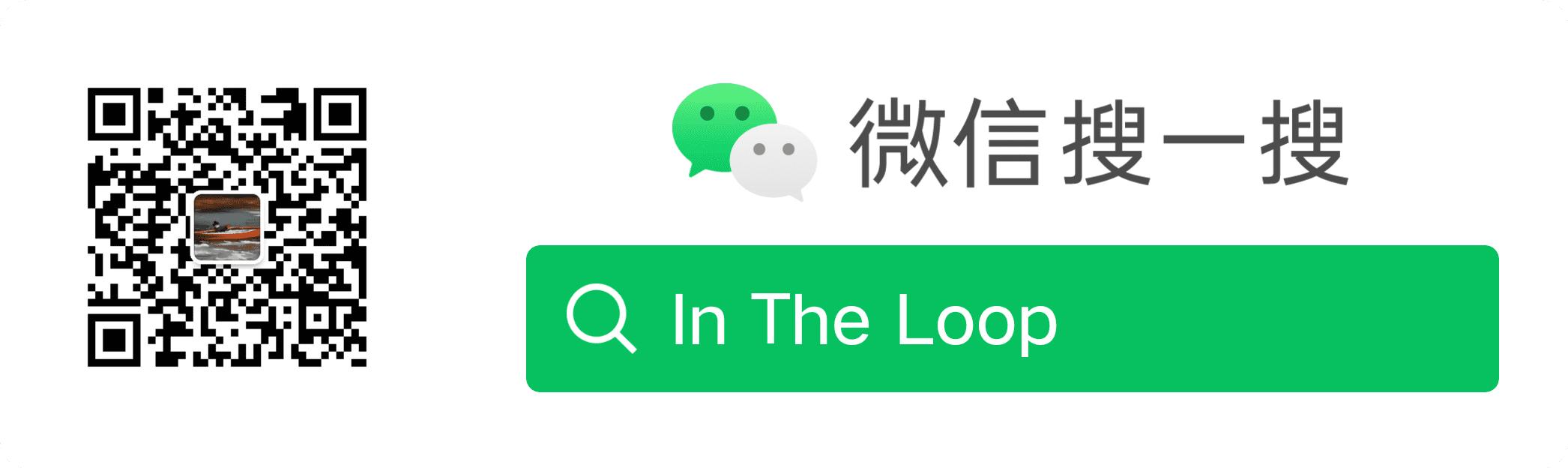